Keywords: Queue
, Stack
, Binary Tree (search, insert, delete, remove, balance)
Usage
See Problem Statement
Problem statements
L
production lines
N
packages
O
Operations
Strategy
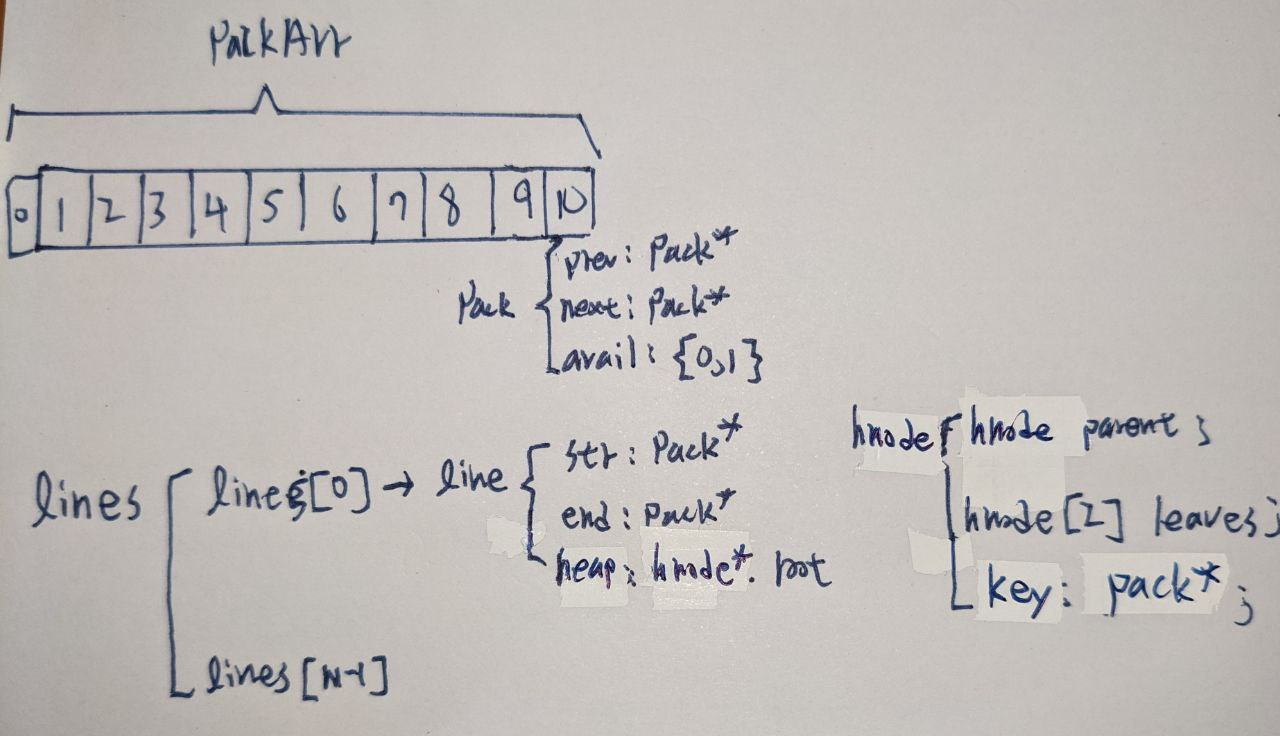
- Production line
- Binomial Max Heap
- Deque
- First-in-First out
- Doubly Linked list ```julia struct int[] array int* next_array int* prev_array end ```
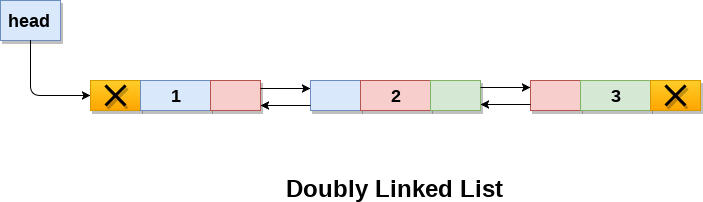
- Peaking a production line
getFirst
: O(1)
getLast
: O(1)
getMax
: O(1)
- Operation
PopFirst
: O(n)
PopLast
: O(n)
PopMax
: O(log n)
- Merge Production line
Queue
Stack
Binary Tree Operation
What is a "balanced" binary tree?
Ref:
AVL Tree
Ref
Time complexity with AVL Tree
Operation | Time |
Search | O(log n) |
Insert | O(log n) |
Delete | O(log n) |
- Advantage of using AVL Tree
- Guarantee: O(log n) for binary search
Ref:
Idea
Hints
- 用 Heap: 快速找到 max, first, last
- Time complexity of merge: <O(n)
Heap Operations
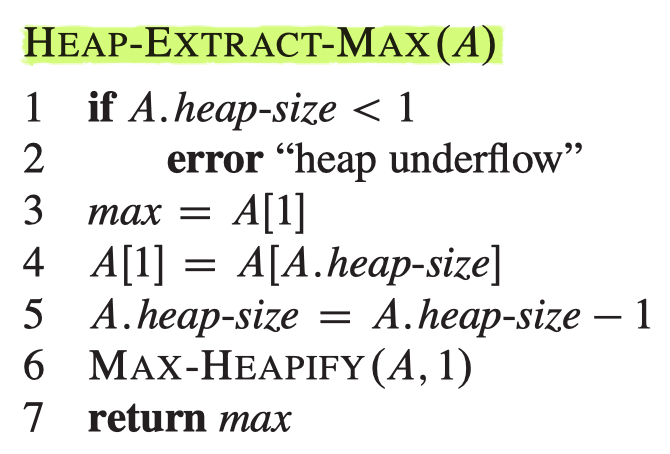
Free a binary tree
deallocate (node):
//do nothing if passed a non-existent node
if node is null
return
//now onto the recursion
deallocate(left node)
deallocate(right node)
free node
Ref: stackoverflow
Returning a void function
Ref: web
Function pointer
#include <stdio.h>
void fun(int a)
{
printf("Value of a is %d\n", a);
}
int main()
{
void (*fun_ptr)(int) = fun;
fun_ptr(10);
return 0;
}
Ref: GreekforGeek
Array of function pointers
int PeekFirstPack(packData, int i)
Definition: list.c:152
int PeekLastPack(packData, int i)
Definition: list.c:173
What are Mutually Dependent Structures in C?
Leftist tree
Ref:
- https://tmt514.gitbooks.io/2016-09/content/tree-ds/leftist-tree.html
- http://sunmoon-template.blogspot.com/2014/12/leftist-tree.html
- https://www.humblec.com/c-implementation-leftist-tree/
- Tutorial: Leftist Tree
References
- Stack, Queue and Heap. [GitBook] 2.